Feature Summary
This PR adds a comprehensive API that allows grouping of Resource
and Page
elements in the Navigation.
The method to do so range from very simple single line of code additions, to an enhanced version of that with Mapped groups, or simply making a Page
or Resource
itself the top level group element.
Effectivly this feature provides this in two flavors:
- Simple Groups: Those that are grouped into a submenu with no top-level page.
- Dynamic Groups: Those that are grouped into a submenu based on a
Page
or Resource
that implements IsGroupItem
.
Simple Groups:
Any resources that defines a $navigationGroup
property will be grouped by overlapping names.
This should be the menu label text for the group - with proper spaces and capitalization you'd like.
class BlogPostResource extends Resource
{
public static $navigationGroup = 'Content Group';
class ContentPage extends Page
{
public static $navigationGroup = 'Content Group';
So in this example there would be a new Content Group sub menu with both these in it.
Simple Groups with Mapped Resources
The second method mimics how Laravel allows for Eloquent morphMap
to be used. In eloquent this maps long class names into uniform database values - for us it maps non-Page
and non-Resource
groups into a single location.
This method should be seen as an enhancement of the prior one. Since ultimately this is required to control the sort and icon settings for any Simple Groups. This would be something you could add in your AppServiceProvider::register
like this:
Filament::navigationGroupMap([
[
'name' => 'Content',
'sort' => -1,
'menus' => [
'default',
'foobar',
]
],
]);
Note: This could be something most useful for the Filament plugins. For instance when a plugin might want to register a group. In theory this should allow all plugins to use the navigationGroupMap without stepping on each others toes.
Dynamic Groups for Resources:
There are two very simple changes one would need to make a Resource into a Group. Simply adding the IsGroupItem
interface and the ImplicitGroupFromResource
trait. And that should be all they need to do. Like:
use Filament\Resources\Concerns\ImplicitGroupFromResource;
use Filament\View\Concerns\IsGroupItem;
class BlogPostResource extends Resource implements IsGroupItem
{
use ImplicitGroupFromResource;
And with just those added the Resource will now be a top-level Group item.
Then to add another resource to this group do:
class BlogCategoryResource extends Resource
{
public static $navigationGroup = 'Blog Posts';
Dynamic Groups for Pages:
There are two very simple changes one would need to make a Resource into a Group. Simply adding the IsGroupItem
interface and the ImplicitGroupFromResource
trait. And that should be all they need to do. Like:
use Filament\Pages\Concerns\ImplicitGroupFromPage;
use Filament\View\Concerns\IsGroupItem;
class ContentPage extends Page implements IsGroupItem
{
use ImplicitGroupFromPage;
And with just those added the Resource will now be a top-level Group item.
Then to add another resource to this group do:
class BlogTagResource extends Resource
{
public static $navigationGroup = 'Content Page';
Results
Simple Groups:
Closed/Open | Open/Closed
:----------:|:-------:
| 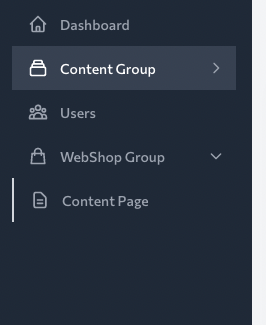
Item within SubMenu Selected
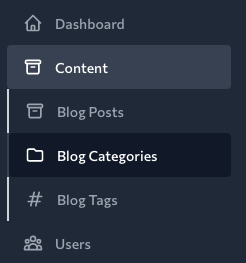
Dynamic Groups:
SubMenu # 1: Blog Posts | SubMenu # 2: Content page
:----------:|:-------:
| 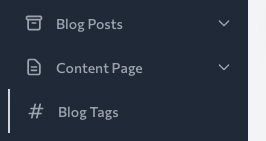
enhancement