@themsaid
So I have seen the previous issues related to this
https://github.com/laravel/telescope/issues/507
https://github.com/laravel/telescope/issues/503
https://github.com/laravel/telescope/issues/411
And I'm suffering the same problem. Some of my jobs are reporting as PENDING even though I know they have completed successfully, and also HORIZON shows the same job as being done correctly.
I've spent a lot of time trying to recreate this using as minimal amount of code as possible, and I hope I've succeeded in providing you with an example that will replicate on your system.
Very quickly as an overall picture.
- I have a LONG running job that gets dispatched.
- Inside that job other events (jobs) get dispatched too, in this case its a broadcast event to update user with some info.
- The LONG running job will always show correct status.
- The broadcast events/jobs do NOT show correct status on TELESCOPE, but DO on horizon.
- If queues are NOT used (ie sync) everything is OK.
- A different queue is used between Long runing job and Broadcast jobs.
Here's some code.
// web.php
Route::get('/test', function () {
dispatch(new \App\Jobs\LongJob());
return "done";
});
// \App\Jobs\LongJob.php
<?php
namespace App\Jobs;
use App\Events\SendUpdate;
use Illuminate\Bus\Queueable;
use Illuminate\Queue\SerializesModels;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
class LongJob implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
public $tries = 1;
public $timeout = 120;
public function handle()
{
sleep(5);
event(new SendUpdate("The first time is:" . time()));
sleep(5);
event(new SendUpdate("The second time is:" . time()));
sleep(10);
}
}
// App\Events\SendUpdate.php
<?php
namespace App\Events;
use Illuminate\Broadcasting\Channel;
use Illuminate\Queue\SerializesModels;
use Illuminate\Broadcasting\PrivateChannel;
use Illuminate\Foundation\Events\Dispatchable;
use Illuminate\Broadcasting\InteractsWithSockets;
use Illuminate\Contracts\Broadcasting\ShouldBroadcast;
class SendUpdate implements ShouldBroadcast
{
use Dispatchable, InteractsWithSockets, SerializesModels;
private $message;
public $broadcastQueue = 'broadcast';
public function __construct($message)
{
$this->message = $message;
}
public function broadcastOn()
{
return new PrivateChannel('App.User.1');
}
}
I have started horizon, and ensured that it is processing jobs from "default" and "broadcast" queues.
Now when I start the whole process job (hitting "/test" in the browser) I'll get the following:
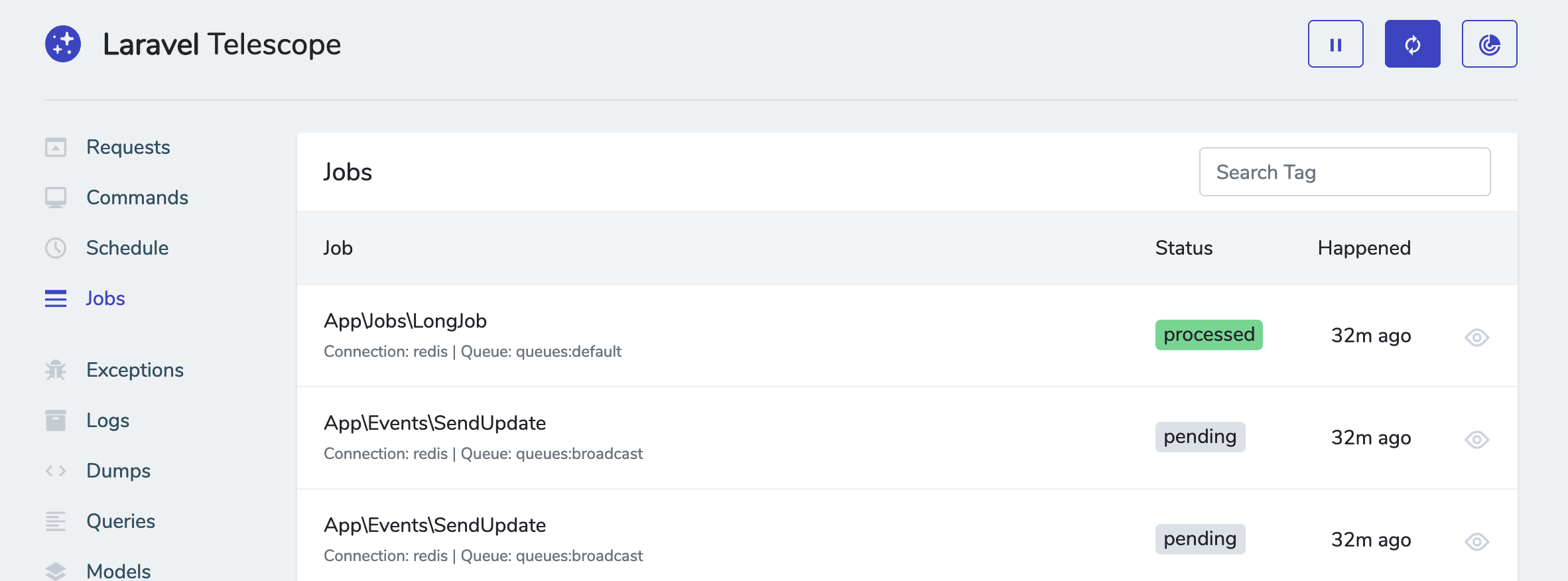
But in horizon it's perfect:
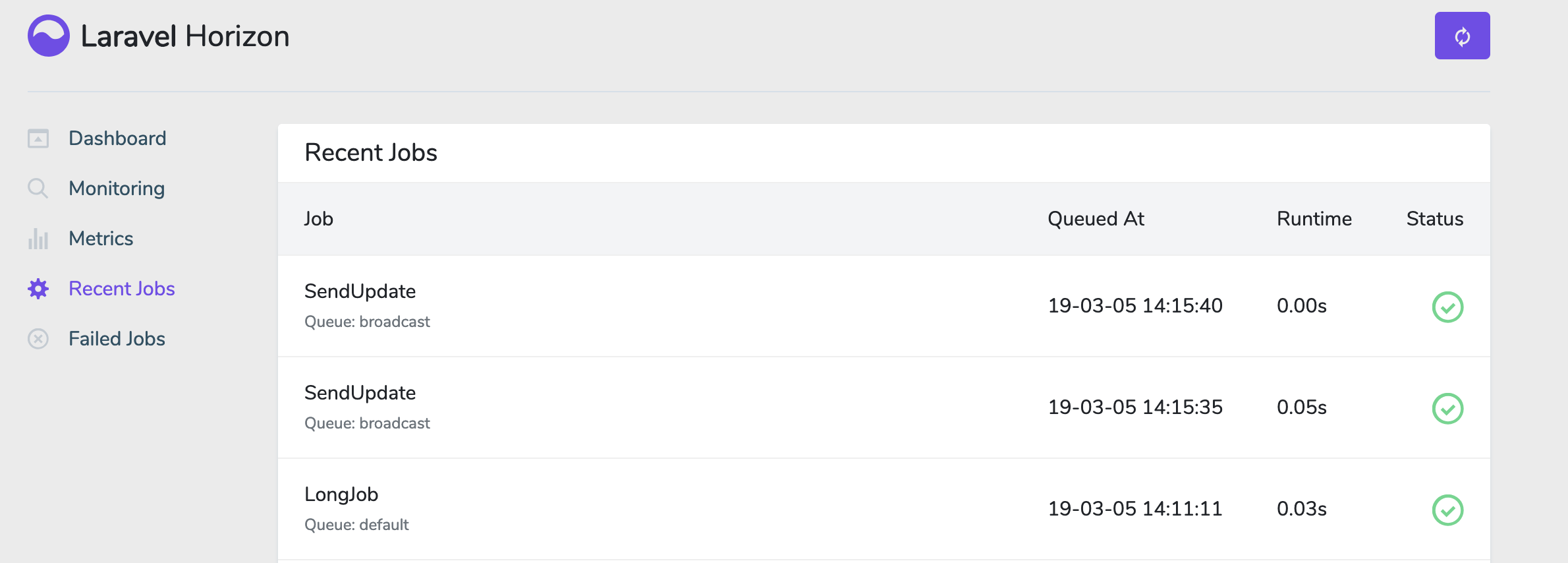
The next post is some info on my debugging attempts.
bug help wanted