This PR adds functionality for Stripe checkout and is a continuation of https://github.com/laravel/cashier-stripe/pull/652. All concerns on the original PR have been resolved since.
Docs: https://github.com/laravel/docs/pull/6465
Usage
Checking out a single priced product:
$checkout = Auth::user()->checkout('price_xxx');
return view('checkout', compact('checkout'));
Checking out a single priced product with a specific quantity:
$checkout = Auth::user()->checkout(['price_xxx' => 5]);
return view('checkout', compact('checkout'));
Checking out multiple priced products and optionally assign quantities to some:
$checkout = Auth::user()->checkout(['price_xxx' => 5, 'price_yyy']);
return view('checkout', compact('checkout'));
Checking out a single priced product and allow promotional codes to be applied:
$checkout = Auth::user()->allowPromotionCodes()->checkout('price_xxx');
return view('checkout', compact('checkout'));
Checking out a new $12 priced product (this will create a new product in the dashboard):
$checkout = Auth::user()->checkoutCharge(1200, 'T-shirt');
return view('checkout', compact('checkout'));
Checking out a new $12 priced product together with a Price ID product:
$checkout = Auth::user()->checkout([
'price_xxx',
[
'price_data' => [
'currency' => Auth::user()->preferredCurrency(),
'product_data' => [
'name' => 'T-Shirt',
],
'unit_amount' => 1200,
],
],
]);
return view('checkout', compact('checkout'));
Check out a subscription:
$checkout = Auth::user()->newSubscription('default', 'price_xxx')->checkout();
return view('checkout', compact('checkout'));
Check out a subscription and allow promotional codes to be applied:
$checkout = Auth::user()->newSubscription('default', 'price_xxx')
->allowPromotionCodes()
->checkout();
return view('checkout', compact('checkout'));
Then place any of these in a view:
{!! $checkout->button() !!}
Button Styling
By default a generated checkout button will have the following styling:
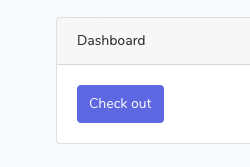
It's however easy to style this. Pass either a custom style
or class
attribute:
{!! $checkout->button('Buy', ['class' => 'text-white bg-blue-500 p-4']) !!}
Todos
- [x] Subscribing to plans
- [x] Charging products
- [x] Single charges
- [x] Webhooks
- [x] Style button
- [x] Tests
- [x] Write documentation
Closes https://github.com/laravel/cashier-stripe/issues/637